Java multidimensional array
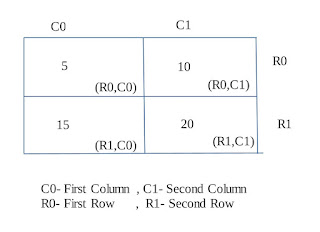
In Java , a multidimensional array data is stored in row and column based index. In the Java programming language, a multidimensional array is an array whose components are themselves arrays. Example : class TwoDArrayDemo{ public static void main(String args[]){ //declaring and initializing 2D array int arr[][] ={{5,10},{15,20}}; //printing 2D array for(int i=0;i<2;i++){ // Row for(int j=0;j<2;j++){ // Column System.out.print("["+i+"]["+j+"] : "+ arr[i][j] +" "); } System.out.println(); } } } Output : [0][0] : 5 [0][1] : 10 [1][0] : 15 [1][1] : 20 Find us : Facebook : @apnaandroid Google+ : Apna Java Youtube : Android & Java Tu