Exception in java
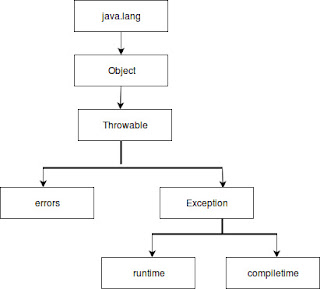
An exception is an event that occurs during the execution of a program that disrupts the normal flow of instructions. Exceptions are two types , one is runtime exception (occurs at runtime) and another one is compile time (occurs in compile time) . Runtime exceptions are as follows : DivideByZeroException NullPointerException ArithmeticException ArrayIndexOutOfBoundsException NumberFormatException etc Compile time exception is Syntax error Example of NullPointerException : public class HelloWorld { public static void main(String[] args) { String str = null ; System.out.println(" String length : "+ str.length() ); } } Result: Exception in thread "main" java.lang.NullPointerException at HelloWorld.main(HelloWorld.java: 6 ) Example of DivideByZeroException : public class HelloWorld { public static void main(String[] args)